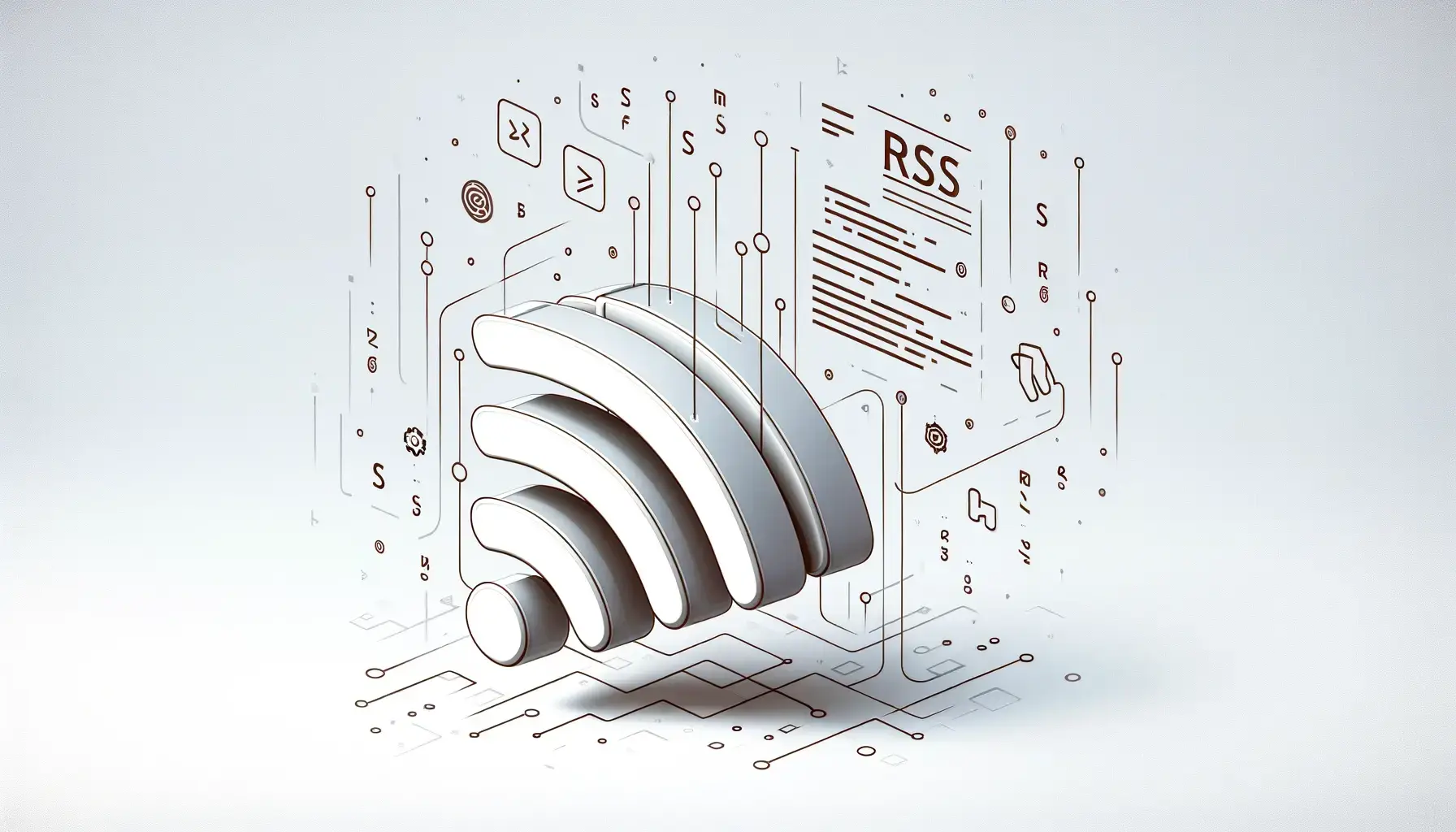
Implementing RSS Feeds in Laravel: A Guide to Boosting Traffic with Google Discover
RSS feeds might seem like a blast from the past, but they’re incredibly relevant today, especially when considering their impact on platforms like Google Discover. Google Discover has the potential to drive significant traffic to your website, making the implementation of an RSS feed a smart strategy.
In the world of Laravel, setting up an RSS feed is surprisingly straightforward, thanks to a handy package from Spatie. Here’s how you can get started:
composer require spatie/laravel-feed
php artisan feed:install
This set of commands will install the necessary Spatie package in your Laravel project.
Now, here’s a twist - instead of following the documented method using eloquent methods, why not streamline the process? We’ll do this by creating a new class for each of our feeds. This approach keeps our models clean, separating feed logic from our main application logic.
We’ll need two main classes - one for the feed and another for each feed item. Let’s take a look at how these classes might be structured.
#Implementing the Feed class
The Feed
class could look something like this:
class Feed {
public function __construct (
protected LanguagesIndex $languages_index
) {}
public function getFeedItems (string $language) {
$language = $this->languages_index->makeFromCode($language);
$article_translations = $this->getArticleTranslations(
Article::query(),
$language
);
return $article_translations->map(function (ArticleTranslation $article_translation) {
return new FeedItemArticle($article_translation);
})->values();
}
}
This class is responsible for gathering and processing the feed items. Notice how it uses a language index to handle translations, ensuring your feed is accessible to a broader audience.
#Implementing the Feedable interface class
Next, the FeedItemArticle
class represents each item in the feed:
class FeedItemArticle implements Feedable {
public function __construct (
protected ArticleTranslation $article_translation
) {}
public function toFeedItem (): FeedItem {
return FeedItem::create()
->id($this->article_translation->slug)
->title($this->article_translation->title)
->summary($this->article_translation->excerpt)
->updated($this->article_translation->published_at)
->authorName('Thorsten')
->link(
route('articles.view.get', [
'language' => $this->article_translation->language,
'slug' => $this->article_translation->slug
])
);
}
}
Each feed item is a standalone entity, encapsulating all necessary data to be displayed in the RSS feed.
Lastly, some tweaks to the config/feed.php
are necessary to get everything up and running:
[...]
'items' => [\App\Domain\Articles\Support\Feed::class, 'getFeedItems', 'language' => 'en'],
'url' => '/feed',
'title' => 'InTheMakings.com',
'description' => 'RSS Feed of inthemakings.com',
[...]
With these changes, your Laravel application will be set to syndicate content effectively, leveraging the power of RSS feeds to attract new visitors via Google Discover.
Remember, keeping up with modern web practices isn’t just about staying trendy; it’s about finding smart, effective ways to engage and expand your audience. And sometimes, that means revisiting old tools like RSS feeds and using them in new, innovative ways.