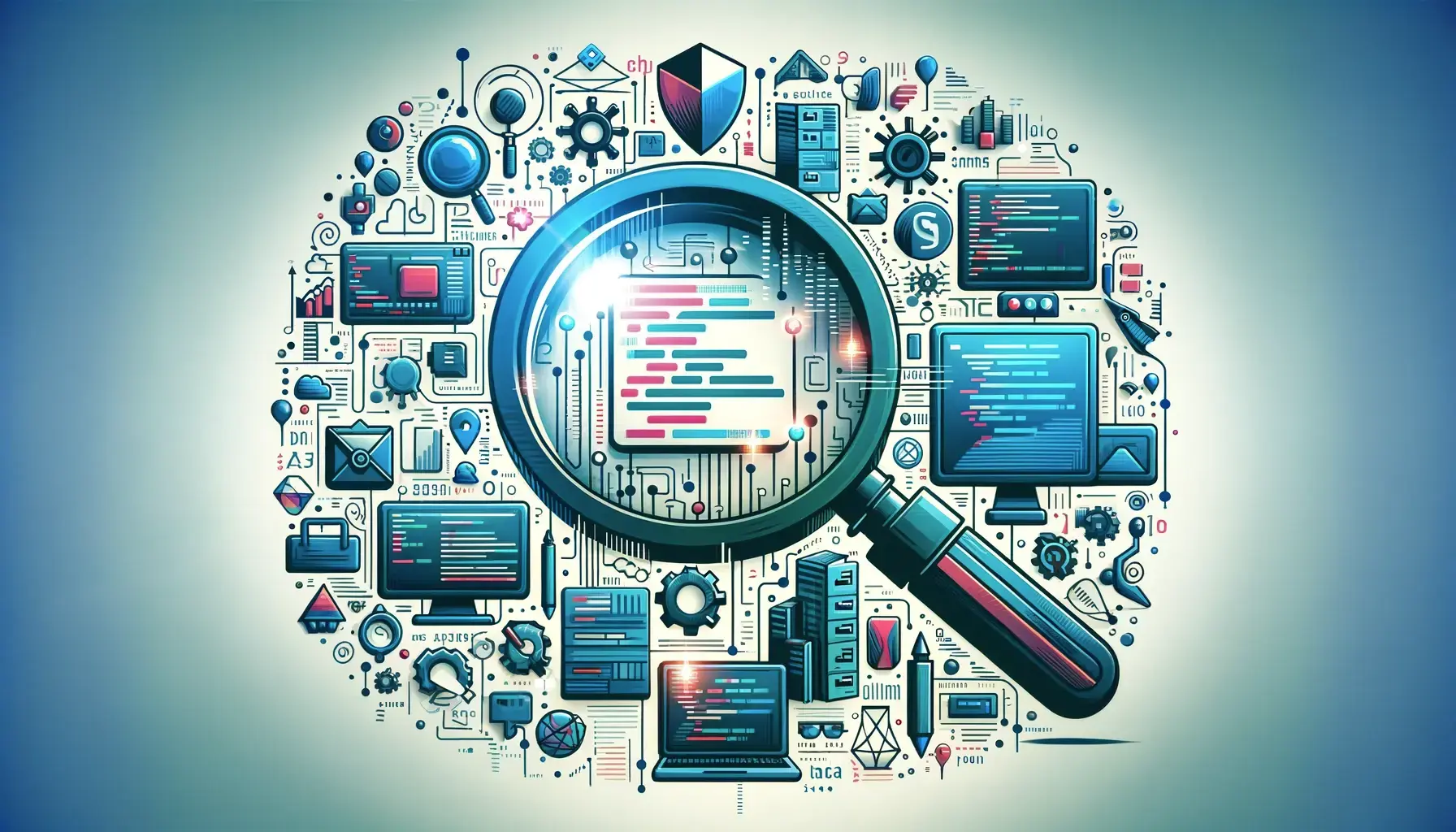
Implementing a Search Feature in Laravel: From Basic to Advanced
Sections
Today, we want to enhance our blog with a search feature, using Laravel. Our approach is methodical and progressive, starting from a simple implementation and gradually moving towards more sophisticated techniques. Let’s dive into the first phase of our implementation.
#Implementation 1: Naive MySQL Search
The initial step in our journey is the Naive MySQL Search. This method is straightforward and quick to implement, making it a great starting point.
#Pros
- Simplicity: It’s incredibly easy and quick to set up.
- No Extra Dependencies: This method doesn’t require any additional software or libraries.
- Cost-Effective: There are no additional costs involved, as it uses existing MySQL capabilities.
#Cons
- Performance Issues: It can be slow, especially with large datasets.
- Limited Search Capabilities: This method only supports exact matches. It lacks advanced features like fuzzy search, synonyms, or stemming.
#Code
Here’s a glimpse of what the implementation could look like in Laravel:
$search = $request->get('q');
$query = Article::query()->whereHas('article_translations', function ($query) use ($search) {
$search_escaped = str_replace('%', '\%', $search);
$query->where('title', 'LIKE', '%' . $search_escaped . '%')
->orWhere('content', 'LIKE', '%' . $search_escaped . '%');
});
#Note
An important aspect to consider, depending on your application’s requirements, is to escape the wildcard character %
in your search string. Failing to do so might allow a user to enter %
as a search term and retrieve all results, which could lead to performance issues or unintended data exposure.
Stay tuned as we continue to evolve our search feature, introducing more advanced methods for better performance and user experience. Happy coding!