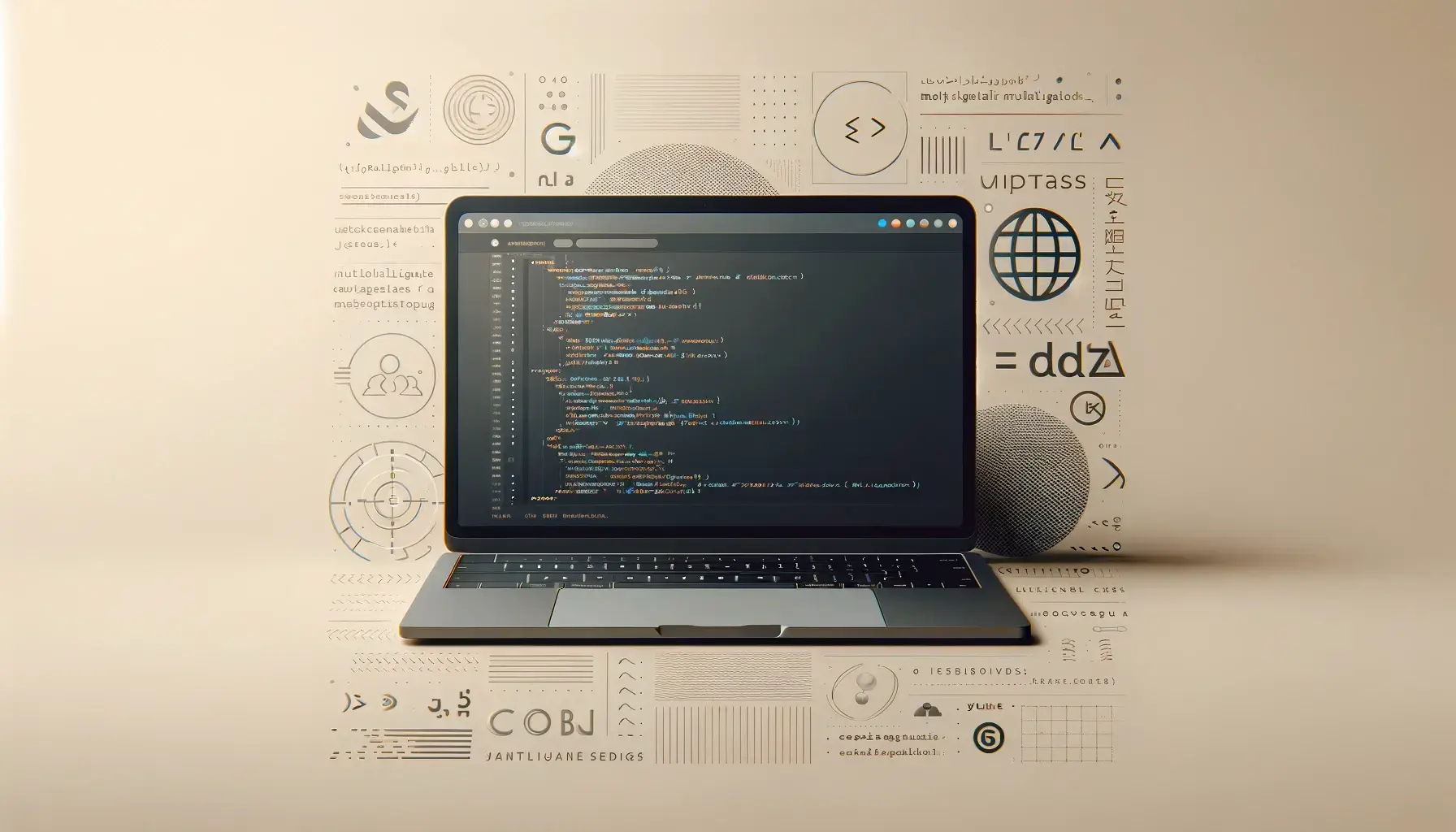
In the Makings: Building Our Tech Blog Step by Step (Part 1)
Sections
Welcome to the first entry of our blog “In the Makings”! This isn’t just an introduction, but a real-time documentation of how we’re crafting this blog from scratch. Think of it as a blend of a plan, a work log, and some tech musings.
#Features: Sketching the Blueprint
Let’s start with the basics. Our blog needs a few essential features:
- A Home Base: A homepage, or index page, displaying all our articles.
- Individual Article Pages: Each piece of content deserves its own space.
- Article Tags: For easy navigation and topic grouping.
- Static Pages: These include necessities like site notice and data privacy policies.
- Bilingual Support: Catering to both English and German speakers, with select articles exclusively in German complementing our German podcast.
- Analytics Insight: We’re opting for fathom for some lightweight tracking.
But wait, there’s more in the pipeline for later:
- Search Functionality: To easily find what you’re looking for.
- A Content Management System (CMS): For now, we’re good with manual article creation.
- A Comment System: To foster community discussions.
- Enhanced SEO: We’ll add more features like schemas, semantic markup, and RSS feeds later on.
#The Base Tech
For the tech enthusiasts, here’s what we’re using:
- Framework: Laravel 10.
- UI Components: daisyUI, for a sleek user interface.
#Database Structure: Laying the Foundations
Our database structure is straightforward but might evolve. For now, it looks like this:
articles
table with basic identifiers and timestamps.article_translations
table for managing multilingual content.tags
table to categorize articles.article_to_tag
table, linking articles to their respective tags.
articles
- id, created_at, updated_at
article_translations
- id, article_id, language, title, slug, content, excerpt, meta_description, indexed_at, published_at
tags
- id, name, slug
article_to_tag
- id, article_id, tag_id
#Routes and Controllers: Mapping the Journey
We’ve mapped out essential routes and their corresponding controllers:
- Root URL for language detection.
- Main and individual article pages.
- Tag-based article listing.
- Search functionality.
- Static pages like imprint and privacy policies.
GET / -> LanguageController@get_detect
GET /{language} -> ArticlesController@get_index
GET /{language}/article/{slug} -> ArticlesController@get_view
GET /{language}/tag/{slug} -> ArticlesController@get_tag
GET /{language}/search -> ArticlesController@get_search
GET /{language}/imprint -> PagesController@get_imprint
GET /{language}/privacy -> PagesController@get_privacy
#Multi-Language Support: Bridging Language Gaps
We store the language in the URL. Laravel helps us detect and redirect to the appropriate language version. But we’re mindful of our German audience who might want to read English articles, and we’re implementing a fallback mechanism for them.
Our language classes in PHP look like this:
- An abstract
Language
class with basic methods. - Specific classes like
GermanLanguage
andEnglishLanguage
. - A
showsFallbacks
function to handle unavailable translations.
class LanguageController extends Controller {
public function get_detect (Request $request) {
$languages = [
'en', 'de'
];
$language = $request->getPreferredLanguage($languages);
return redirect()->route('articles.index.get', ['language' => $language]);
}
}
app/Domain/Language/Support/
Language.php
EnglishLanguage.php
GermanLanguage.php
LanguagesIndex.php
A language is class is built like this:
abstract class Language {
abstract public static function getCode (): string;
abstract public static function showsFallbacks (): array;
final public function __toString (): string {
return static::getCode();
}
}
An implementation of a language looks like this:
class GermanLanguage extends Language {
public static function getCode (): string {
return 'de';
}
public static function showsFallbacks (): array {
return [EnglishLanguage::class];
}
}
#Writing with Markdown: Keeping It Simple
Markdown is our choice for writing articles, thanks to its simplicity. We’re using parsedown, a top-notch markdown parser for PHP. Integrating it into our ArticlesController
is straightforward and efficient:
public function get_view (string $language, string $slug) {
[...]
$parsedown = new \Parsedown();
$html = $parsedown->text($article_translation->content);
[...]
}
That wraps up our initial blueprint! We’re excited to build this blog and share our journey with you. Stay tuned for more updates and tech insights as we progress. 🚀