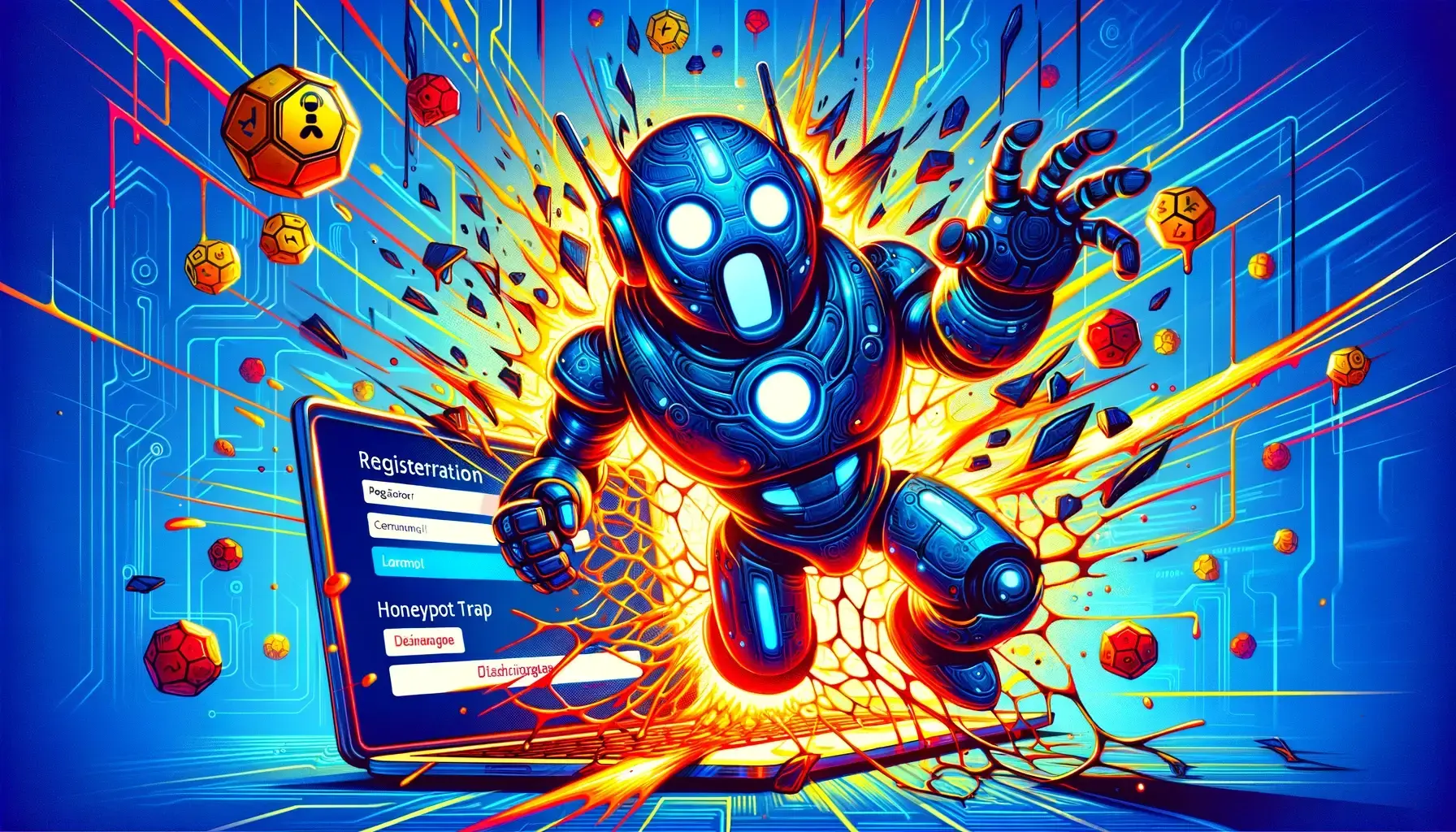
Reducing Bot Spam in Laravel
Sections
Today, we’re tackling a problem that’s been bugging us for a while: bot registrations. You know, those automated nuisances that sign up on your website but aren’t real people? Yeah, those. Our site has been getting about 15 bot registrations daily, which is quite a headache. So, we’ve decided to try out some solutions, ranging from simple ones to more complex tactics, and see how each one impacts our bot registration numbers.
#Measure 1: Naive Honeypot
First up is something called a “honeypot.” Now, this isn’t about attracting bees or bears. In the tech world, a honeypot is a clever little trap. It’s a form field on your website that’s invisible to human users but visible to bots. If this field gets filled out, it’s a dead giveaway that a bot’s at work.
Our registration page is pretty straightforward, asking only for an email address and a password. Our honeypot? A “name” field that’s hidden from human eyes but a tempting target for bots. Here’s how I set it up:
<input type="text" name="name" id="name" class="form-control hidden" autocomplete="off" tabindex="-1">
And in our controller, we handle it like this:
$request->validate([
'email' => 'required|email|unique:users,email',
'password' => 'required|string|min:6',
// Honeypot
'name' => 'nullable|string',
]);
if ($request->get('name') !== null) {
return redirect()->route('front.home.get');
}
Pretty straightforward, right? We’re setting this up and will give it a week to see how it performs. Stay tuned for an update on whether this naive honeypot helps reduce those annoying bot registrations. Catch you in the next post!
#Update after 1 week deployed
Great news! Our naive honeypot has effectively prevented all bot registrations. However, there’s an unexpected twist. Instead of submitting the form just once, bots are now trying three times every time they’re caught. This results in us receiving a flood of notifications each time a bot registration is thwarted. This is a new behavior that we haven’t encountered before.
To tackle this, we’re considering adding a random delay to the honeypot. The idea is that by slowing down the response, we might confuse the bots, making them less likely to realize their registration attempt failed. Here’s the code snippet for that:
// add random delay to prevent bots from knowing that the registration failed
sleep(rand(2, 4));
Let’s see if this tweak makes our honeypot even more effective. We’ll keep you updated on our battle against bot registrations. Stay tuned!