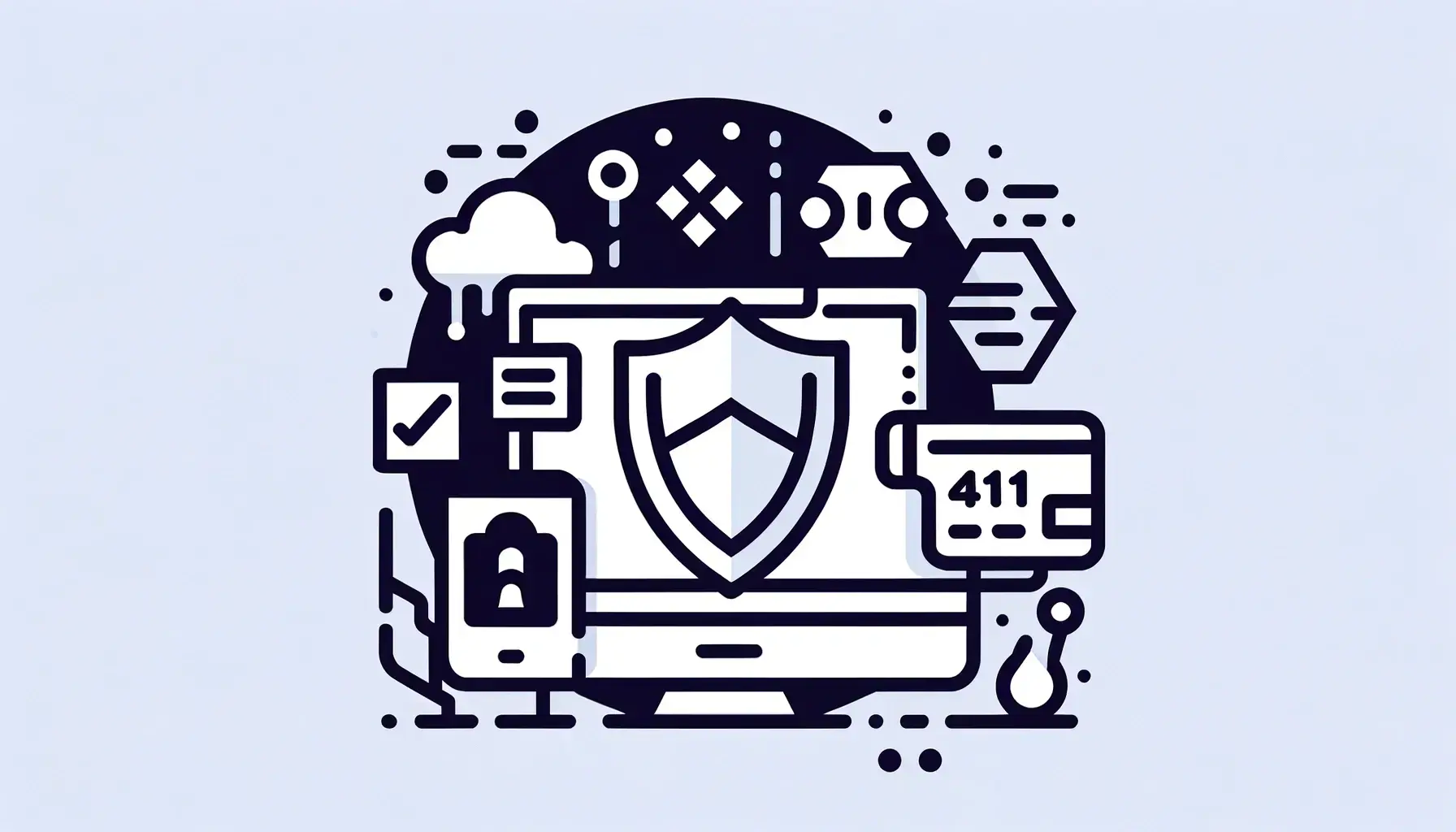
CSRF Tokens: Solving the '419 Page Expired' Error in Laravel
Sections
Today, we’re diving into a common but often misunderstood issue in Laravel: the “419 Page Expired” error. This error ties into how Laravel handles security, specifically through something called a CSRF token. Let’s unravel this mystery together!
#What’s a CSRF Token, and Why is it Important?
CSRF stands for Cross-Site Request Forgery. Imagine you’re logged into a website and, unbeknownst to you, visit a malicious site. This bad actor could secretly make requests to the website where you’re logged in, potentially changing information like your email address. Scary, right?
This is where CSRF tokens play hero. They are random strings generated by your server and embedded in forms. When you submit a form, the server verifies this token. If it doesn’t match what it expects, it rejects the request, effectively blocking the bad actor’s play.
#Common Missteps in Handling CSRF Tokens
Some solutions suggest using the $except = []
array in the VerifyCsrfToken
middleware to exclude routes from CSRF token checks. This is risky! It’s like leaving your house door unlocked. Sure, you trust your neighbors, but what if someone else walks in?
Also, Laravel’s default handling of a CSRF token mismatch isn’t exactly user-friendly. It redirects to a “419 Page Expired” page, leaving users confused and often stuck in a loop if they try to go back and resubmit the form.
#The Right Way to Handle CSRF Token Mismatch
Our goal? Redirect users back to the original page with a helpful message, while also generating a fresh CSRF token to maintain security. Here’s how you can do it:
In your Exception handler, which you will find at app/Exceptions/Handler.php
, add this code:
class Handler extends ExceptionHandler
{
...
public function register(): void
{
...
// Redirect back on TokenMismatchException
$this->renderable(function (\Symfony\Component\HttpKernel\Exception\HttpException $e) {
if ($e->getPrevious() instanceof \Illuminate\Session\TokenMismatchException) {
return redirect()
->back()
// Don't include sensitive data in the session
->exceptInput('password', 'password_confirmation')
// Add an error message
->withErrors(['token' => 'Session expired, please try again']);
}
});
}
}
To display this error message in your Blade template, add:
@if ($errors->has('token'))
<div class="alert alert-danger">
{{ $errors->first('token') }}
</div>
@endif
And voilà! Users are now redirected back with a clear message, and a new CSRF token is ready for their next attempt.
By understanding and implementing this solution, you can enhance the user experience and maintain robust security in your Laravel applications. Remember, the tech world is full of intricate details; understanding them is key to crafting seamless, secure digital experiences.
Until next time, keep coding and stay curious! 🚀