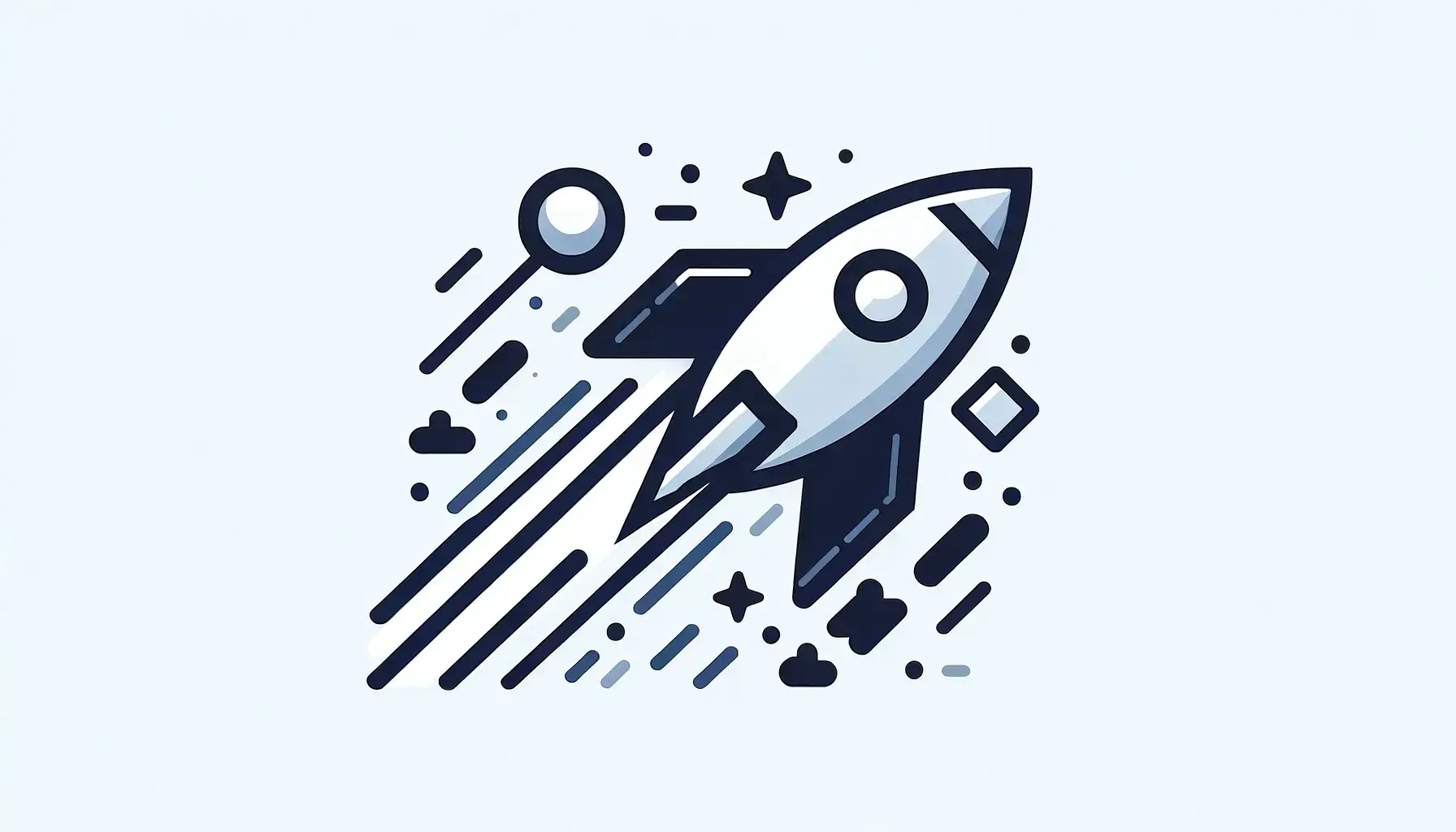
Laravel Performance Tweaks
Laravel stands out as a popular PHP framework known for its elegant syntax and rich features. However, like any powerful tool, its misuse can lead to performance issues. In this article, we’ll explore practical tweaks to enhance Laravel’s performance. Remember, this is an evolving list, and we’ll update it with new insights over time.
#Making Implicit Runtime Laravel Magic Explicit:
Laravel’s “magic” often simplifies coding, but sometimes it’s better to be explicit, especially for performance. Let’s dive into some examples:
#Specifying the $table
Property:
In Laravel, model classes don’t require you to explicitly set the table they represent. But, defining it can avoid the runtime cost of guessing the table name.
For instance, in a User
model:
class User extends Authenticatable
{
protected $table = 'users';
}
Here, we explicitly define that the User
model is tied to the users
table. This small step can improve the performance by reducing the computation required to infer the table name.
#Optimizing Relationships with BelongsTo
:
Relationships are a core feature of Laravel, allowing models to interact with each other. When defining a BelongsTo
relationship, it’s more efficient to specify all keys rather than letting Laravel guess them.
For example, instead of:
public function user(): BelongsTo {
return $this->belongsTo(User::class);
}
Do this:
public function user(): BelongsTo {
return $this->belongsTo(User::class, 'user_id', 'id', 'user');
}
In the above snippet, we explicitly define the foreign key (user_id
), the owner key (id
), and the relation name (user
). This clarity prevents Laravel from making runtime calculations to determine these parameters.
#Understanding the Underlying Mechanism:
To appreciate why this matters, let’s peek into the belongsTo
method:
public function belongsTo($related, $foreignKey = null, $ownerKey = null, $relation = null)
{
// ...
if (is_null($relation)) {
$relation = $this->guessBelongsToRelation();
}
// ...
if (is_null($foreignKey)) {
$foreignKey = Str::snake($relation).'_'.$instance->getKeyName();
}
// ...
$ownerKey = $ownerKey ?: $instance->getKeyName();
// ...
}
This method, part of Laravel’s Eloquent ORM, uses conditional logic to infer missing parameters. If we don’t specify these parameters, Laravel uses string manipulation and naming conventions to guess them, which, though convenient, adds overhead to each database query. By being explicit, we save Laravel from doing this extra work, leading to faster database interactions.
In summary, being explicit with your Laravel models and relationships can significantly enhance performance. It’s a simple yet effective tweak that ensures smoother and more efficient database interactions. Stay tuned for more tips and updates in the world of Laravel optimization!